mirror of
https://github.com/gohugoio/hugo.git
synced 2024-06-27 21:25:07 +00:00
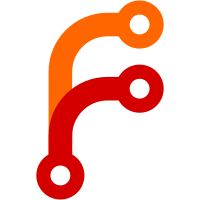
A sample config: ```toml defaultContentLanguage = "en" defaultContentLanguageInSubdir = true [Languages] [Languages.en] weight = 10 title = "In English" languageName = "English" contentDir = "content/english" [Languages.nn] weight = 20 title = "På Norsk" languageName = "Norsk" contentDir = "content/norwegian" ``` The value of `contentDir` can be any valid path, even absolute path references. The only restriction is that the content dirs cannot overlap. The content files will be assigned a language by 1. The placement: `content/norwegian/post/my-post.md` will be read as Norwegian content. 2. The filename: `content/english/post/my-post.nn.md` will be read as Norwegian even if it lives in the English content folder. The content directories will be merged into a big virtual filesystem with one simple rule: The most specific language file will win. This means that if both `content/norwegian/post/my-post.md` and `content/english/post/my-post.nn.md` exists, they will be considered duplicates and the version inside `content/norwegian` will win. Note that translations will be automatically assigned by Hugo by the content file's relative placement, so `content/norwegian/post/my-post.md` will be a translation of `content/english/post/my-post.md`. If this does not work for you, you can connect the translations together by setting a `translationKey` in the content files' front matter. Fixes #4523 Fixes #4552 Fixes #4553
87 lines
1.9 KiB
Go
87 lines
1.9 KiB
Go
// Copyright 2017-present The Hugo Authors. All rights reserved.
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
package hugolib
|
|
|
|
import (
|
|
"fmt"
|
|
"io"
|
|
"os"
|
|
"strings"
|
|
|
|
"github.com/spf13/afero"
|
|
)
|
|
|
|
// GC requires a build first.
|
|
func (h *HugoSites) GC() (int, error) {
|
|
s := h.Sites[0]
|
|
fs := h.PathSpec.BaseFs.ResourcesFs
|
|
|
|
imageCacheDir := s.resourceSpec.GenImagePath
|
|
if len(imageCacheDir) < 10 {
|
|
panic("invalid image cache")
|
|
}
|
|
|
|
isInUse := func(filename string) bool {
|
|
key := strings.TrimPrefix(filename, imageCacheDir)
|
|
for _, site := range h.Sites {
|
|
if site.resourceSpec.IsInCache(key) {
|
|
return true
|
|
}
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
counter := 0
|
|
|
|
err := afero.Walk(fs, imageCacheDir, func(path string, info os.FileInfo, err error) error {
|
|
if info == nil {
|
|
return nil
|
|
}
|
|
|
|
if !strings.HasPrefix(path, imageCacheDir) {
|
|
return fmt.Errorf("Invalid state, walk outside of resource dir: %q", path)
|
|
}
|
|
|
|
if info.IsDir() {
|
|
f, err := fs.Open(path)
|
|
if err != nil {
|
|
return nil
|
|
}
|
|
defer f.Close()
|
|
_, err = f.Readdirnames(1)
|
|
if err == io.EOF {
|
|
// Empty dir.
|
|
s.Fs.Source.Remove(path)
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
inUse := isInUse(path)
|
|
if !inUse {
|
|
err := fs.Remove(path)
|
|
if err != nil && !os.IsNotExist(err) {
|
|
s.Log.ERROR.Printf("Failed to remove %q: %s", path, err)
|
|
} else {
|
|
counter++
|
|
}
|
|
}
|
|
return nil
|
|
})
|
|
|
|
return counter, err
|
|
|
|
}
|