mirror of
https://github.com/gohugoio/hugo.git
synced 2024-07-02 15:20:28 +00:00
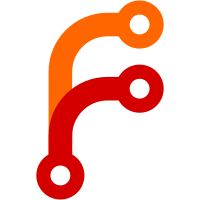
This commit moves almost all of the template functions into separate packages under tpl/ and adds a namespace framework. All changes should be backward compatible for end users, as all existing function names in the template funcMap are left intact. Seq and DoArithmatic have been moved out of the helpers package and into template namespaces. Most of the tests involved have been refactored, and many new tests have been written. There's still work to do, but this is a big improvement. I got a little overzealous and added some new functions along the way: - strings.Contains - strings.ContainsAny - strings.HasSuffix - strings.TrimPrefix - strings.TrimSuffix Documentation is forthcoming. Fixes #3042
128 lines
3.3 KiB
Go
128 lines
3.3 KiB
Go
// Copyright 2015 The Hugo Authors. All rights reserved.
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
package hugolib
|
|
|
|
import (
|
|
"reflect"
|
|
"sort"
|
|
"sync"
|
|
|
|
"github.com/spf13/hugo/tpl/math"
|
|
)
|
|
|
|
// Scratch is a writable context used for stateful operations in Page/Node rendering.
|
|
type Scratch struct {
|
|
values map[string]interface{}
|
|
mu sync.RWMutex
|
|
}
|
|
|
|
// Add will, for single values, add (using the + operator) the addend to the existing addend (if found).
|
|
// Supports numeric values and strings.
|
|
//
|
|
// If the first add for a key is an array or slice, then the next value(s) will be appended.
|
|
func (c *Scratch) Add(key string, newAddend interface{}) (string, error) {
|
|
|
|
var newVal interface{}
|
|
c.mu.RLock()
|
|
existingAddend, found := c.values[key]
|
|
c.mu.RUnlock()
|
|
if found {
|
|
var err error
|
|
|
|
addendV := reflect.ValueOf(existingAddend)
|
|
|
|
if addendV.Kind() == reflect.Slice || addendV.Kind() == reflect.Array {
|
|
nav := reflect.ValueOf(newAddend)
|
|
if nav.Kind() == reflect.Slice || nav.Kind() == reflect.Array {
|
|
newVal = reflect.AppendSlice(addendV, nav).Interface()
|
|
} else {
|
|
newVal = reflect.Append(addendV, nav).Interface()
|
|
}
|
|
} else {
|
|
newVal, err = math.DoArithmetic(existingAddend, newAddend, '+')
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
}
|
|
} else {
|
|
newVal = newAddend
|
|
}
|
|
c.mu.Lock()
|
|
c.values[key] = newVal
|
|
c.mu.Unlock()
|
|
return "", nil // have to return something to make it work with the Go templates
|
|
}
|
|
|
|
// Set stores a value with the given key in the Node context.
|
|
// This value can later be retrieved with Get.
|
|
func (c *Scratch) Set(key string, value interface{}) string {
|
|
c.mu.Lock()
|
|
c.values[key] = value
|
|
c.mu.Unlock()
|
|
return ""
|
|
}
|
|
|
|
// Get returns a value previously set by Add or Set
|
|
func (c *Scratch) Get(key string) interface{} {
|
|
c.mu.RLock()
|
|
val := c.values[key]
|
|
c.mu.RUnlock()
|
|
|
|
return val
|
|
}
|
|
|
|
// SetInMap stores a value to a map with the given key in the Node context.
|
|
// This map can later be retrieved with GetSortedMapValues.
|
|
func (c *Scratch) SetInMap(key string, mapKey string, value interface{}) string {
|
|
c.mu.Lock()
|
|
_, found := c.values[key]
|
|
if !found {
|
|
c.values[key] = make(map[string]interface{})
|
|
}
|
|
|
|
c.values[key].(map[string]interface{})[mapKey] = value
|
|
c.mu.Unlock()
|
|
return ""
|
|
}
|
|
|
|
// GetSortedMapValues returns a sorted map previously filled with SetInMap
|
|
func (c *Scratch) GetSortedMapValues(key string) interface{} {
|
|
c.mu.RLock()
|
|
|
|
if c.values[key] == nil {
|
|
c.mu.RUnlock()
|
|
return nil
|
|
}
|
|
|
|
unsortedMap := c.values[key].(map[string]interface{})
|
|
c.mu.RUnlock()
|
|
var keys []string
|
|
for mapKey := range unsortedMap {
|
|
keys = append(keys, mapKey)
|
|
}
|
|
|
|
sort.Strings(keys)
|
|
|
|
sortedArray := make([]interface{}, len(unsortedMap))
|
|
for i, mapKey := range keys {
|
|
sortedArray[i] = unsortedMap[mapKey]
|
|
}
|
|
|
|
return sortedArray
|
|
}
|
|
|
|
func newScratch() *Scratch {
|
|
return &Scratch{values: make(map[string]interface{})}
|
|
}
|