mirror of
https://github.com/gohugoio/hugo.git
synced 2024-07-07 01:32:15 +00:00
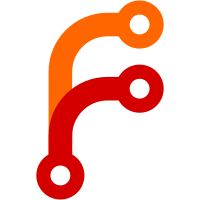
Moving the ugly urls logic to the target. There is still UglyUrl logic in page for the permlink but this is dealing with the generate of urls.
65 lines
1.4 KiB
Go
65 lines
1.4 KiB
Go
package target
|
|
|
|
import (
|
|
"testing"
|
|
)
|
|
|
|
func TestFileTranslator(t *testing.T) {
|
|
tests := []struct {
|
|
content string
|
|
expected string
|
|
}{
|
|
{"/", "index.html"},
|
|
{"index.html", "index/index.html"},
|
|
{"foo", "foo/index.html"},
|
|
{"foo.html", "foo/index.html"},
|
|
{"foo.xhtml", "foo/index.xhtml"},
|
|
{"section/foo", "section/foo/index.html"},
|
|
{"section/foo.html", "section/foo/index.html"},
|
|
{"section/foo.rss", "section/foo/index.rss"},
|
|
}
|
|
|
|
for _, test := range tests {
|
|
f := new(Filesystem)
|
|
dest, err := f.Translate(test.content)
|
|
if err != nil {
|
|
t.Fatalf("Translate returned and unexpected err: %s", err)
|
|
}
|
|
|
|
if dest != test.expected {
|
|
t.Errorf("Tranlate expected return: %s, got: %s", test.expected, dest)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestTranslateUglyUrls(t *testing.T) {
|
|
tests := []struct {
|
|
content string
|
|
expected string
|
|
}{
|
|
{"foo.html", "foo.html"},
|
|
{"/", "index.html"},
|
|
{"index.html", "index.html"},
|
|
}
|
|
|
|
for _, test := range tests {
|
|
f := &Filesystem{UglyUrls: true}
|
|
dest, err := f.Translate(test.content)
|
|
if err != nil {
|
|
t.Fatalf("Translate returned an unexpected err: %s", err)
|
|
}
|
|
|
|
if dest != test.expected {
|
|
t.Errorf("Translate expected return: %s, got: %s", test.expected, dest)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestTranslateDefaultExtension(t *testing.T) {
|
|
f := &Filesystem{DefaultExtension: ".foobar"}
|
|
dest, _ := f.Translate("baz")
|
|
if dest != "baz/index.foobar" {
|
|
t.Errorf("Translate expected return: %s, got %s", "baz/index.foobar", dest)
|
|
}
|
|
}
|