mirror of
https://github.com/gohugoio/hugo.git
synced 2024-07-02 23:30:00 +00:00
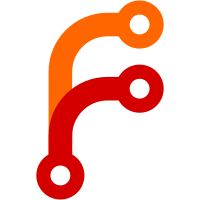
This commit adds the interface ContentReWriter in the tranformer chain. This is backed by two pooled byte buffers, alternating between being the reader or the writer. This keeps the performance characteristic of the old implementation, but in a thread safe way. Fixes #911 Benchmark old vs new: benchmark old ns/op new ns/op delta BenchmarkAbsURL 17614 17384 -1.31% BenchmarkXMLAbsURL 9431 9248 -1.94% benchmark old allocs new allocs delta BenchmarkAbsURL 24 28 +16.67% BenchmarkXMLAbsURL 12 14 +16.67% benchmark old bytes new bytes delta BenchmarkAbsURL 3295 3424 +3.92% BenchmarkXMLAbsURL 1954 1987 +1.69%
82 lines
1.2 KiB
Go
82 lines
1.2 KiB
Go
package transform
|
|
|
|
import (
|
|
"bytes"
|
|
bp "github.com/spf13/hugo/bufferpool"
|
|
"io"
|
|
)
|
|
|
|
type trans func(rw ContentReWriter)
|
|
|
|
type link trans
|
|
|
|
type chain []link
|
|
|
|
func NewChain(trs ...link) chain {
|
|
return trs
|
|
}
|
|
|
|
func NewEmptyTransforms() []link {
|
|
return make([]link, 0, 20)
|
|
}
|
|
|
|
// ContentReWriter is an interface that enables rotation
|
|
// of pooled buffers in the transformer chain.
|
|
type ContentReWriter interface {
|
|
Content() []byte
|
|
io.Writer
|
|
}
|
|
|
|
// Implements ContentReWriter
|
|
// Content is read from the from-buffer,
|
|
// and rewritten to to the to-buffer.
|
|
type fromToBuffer struct {
|
|
from *bytes.Buffer
|
|
to *bytes.Buffer
|
|
}
|
|
|
|
func (ft fromToBuffer) Write(p []byte) (n int, err error) {
|
|
return ft.to.Write(p)
|
|
}
|
|
|
|
func (ft fromToBuffer) Content() []byte {
|
|
return ft.from.Bytes()
|
|
}
|
|
|
|
func (c *chain) Apply(w io.Writer, r io.Reader) error {
|
|
|
|
b1 := bp.GetBuffer()
|
|
defer bp.PutBuffer(b1)
|
|
|
|
b1.ReadFrom(r)
|
|
|
|
if len(*c) == 0 {
|
|
b1.WriteTo(w)
|
|
return nil
|
|
}
|
|
|
|
b2 := bp.GetBuffer()
|
|
defer bp.PutBuffer(b2)
|
|
|
|
fb := &fromToBuffer{from: b1, to: b2}
|
|
|
|
for i, tr := range *c {
|
|
if i > 0 {
|
|
if fb.from == b1 {
|
|
fb.from = b2
|
|
fb.to = b1
|
|
fb.to.Reset()
|
|
} else {
|
|
fb.from = b1
|
|
fb.to = b2
|
|
fb.to.Reset()
|
|
}
|
|
}
|
|
|
|
tr(fb)
|
|
}
|
|
|
|
fb.to.WriteTo(w)
|
|
return nil
|
|
}
|