mirror of
https://github.com/gohugoio/hugo.git
synced 2024-07-02 15:20:28 +00:00
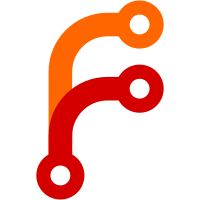
This is needed to make shortcode users happy with the new multilanguage support, but it will also solve many other related posts about "stuff not available in the shortcode". We will have to revisit this re the handler chain at some point, but that will be easier now as the integration test story has improved so much. As part of this commit, the site-building tests in page_test.go is refreshed, they now tests for all the rendering engines (when available), and all of them now uses the same code-path as used in production. Fixes #1229 Fixes #2323 Fixes ##1076
118 lines
2.5 KiB
Go
118 lines
2.5 KiB
Go
// Copyright 2015 The Hugo Authors. All rights reserved.
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
package hugolib
|
|
|
|
import (
|
|
"errors"
|
|
|
|
"fmt"
|
|
|
|
"github.com/spf13/hugo/source"
|
|
)
|
|
|
|
var handlers []Handler
|
|
|
|
type MetaHandler interface {
|
|
// Read the Files in and register
|
|
Read(*source.File, *Site, HandleResults)
|
|
|
|
// Generic Convert Function with coordination
|
|
Convert(interface{}, *Site, HandleResults)
|
|
|
|
Handle() Handler
|
|
}
|
|
|
|
type HandleResults chan<- HandledResult
|
|
|
|
func NewMetaHandler(in string) *MetaHandle {
|
|
x := &MetaHandle{ext: in}
|
|
x.Handler()
|
|
return x
|
|
}
|
|
|
|
type MetaHandle struct {
|
|
handler Handler
|
|
ext string
|
|
}
|
|
|
|
func (mh *MetaHandle) Read(f *source.File, s *Site, results HandleResults) {
|
|
if h := mh.Handler(); h != nil {
|
|
results <- h.Read(f, s)
|
|
return
|
|
}
|
|
|
|
results <- HandledResult{err: errors.New("No handler found"), file: f}
|
|
}
|
|
|
|
func (mh *MetaHandle) Convert(i interface{}, s *Site, results HandleResults) {
|
|
h := mh.Handler()
|
|
|
|
if f, ok := i.(*source.File); ok {
|
|
results <- h.FileConvert(f, s)
|
|
return
|
|
}
|
|
|
|
if p, ok := i.(*Page); ok {
|
|
if p == nil {
|
|
results <- HandledResult{err: errors.New("file resulted in a nil page")}
|
|
return
|
|
}
|
|
|
|
if h == nil {
|
|
results <- HandledResult{err: fmt.Errorf("No handler found for page '%s'. Verify the markup is supported by Hugo.", p.FullFilePath())}
|
|
return
|
|
}
|
|
|
|
results <- h.PageConvert(p, s.Tmpl)
|
|
}
|
|
}
|
|
|
|
func (mh *MetaHandle) Handler() Handler {
|
|
if mh.handler == nil {
|
|
mh.handler = FindHandler(mh.ext)
|
|
|
|
// if no handler found, use default handler
|
|
if mh.handler == nil {
|
|
mh.handler = FindHandler("*")
|
|
}
|
|
}
|
|
return mh.handler
|
|
}
|
|
|
|
func FindHandler(ext string) Handler {
|
|
for _, h := range Handlers() {
|
|
if HandlerMatch(h, ext) {
|
|
return h
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func HandlerMatch(h Handler, ext string) bool {
|
|
for _, x := range h.Extensions() {
|
|
if ext == x {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|
|
|
|
func RegisterHandler(h Handler) {
|
|
handlers = append(handlers, h)
|
|
}
|
|
|
|
func Handlers() []Handler {
|
|
return handlers
|
|
}
|