mirror of
https://github.com/gohugoio/hugo.git
synced 2024-07-13 20:38:00 +00:00
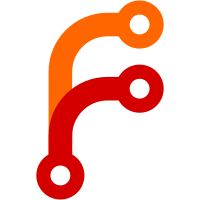
In watch mode it should continue to watch for changes, in any other mode it should exit with a -1 error code so can check for success when scripting
71 lines
2 KiB
Go
71 lines
2 KiB
Go
// Copyright © 2013 Steve Francia <spf@spf13.com>.
|
|
//
|
|
// Licensed under the Simple Public License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
// http://opensource.org/licenses/Simple-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
package commands
|
|
|
|
import (
|
|
"fmt"
|
|
"github.com/spf13/cobra"
|
|
"net/http"
|
|
"strconv"
|
|
)
|
|
|
|
var serverPort int
|
|
var serverWatch bool
|
|
|
|
func init() {
|
|
serverCmd.Flags().IntVarP(&serverPort, "port", "p", 1313, "port to run the server on")
|
|
serverCmd.Flags().BoolVarP(&serverWatch, "watch", "w", false, "watch filesystem for changes and recreate as needed")
|
|
}
|
|
|
|
var serverCmd = &cobra.Command{
|
|
Use: "server",
|
|
Short: "Hugo runs it's own a webserver to render the files",
|
|
Long: `Hugo is able to run it's own high performance web server.
|
|
Hugo will render all the files defined in the source directory and
|
|
Serve them up.`,
|
|
Run: server,
|
|
}
|
|
|
|
func server(cmd *cobra.Command, args []string) {
|
|
InitializeConfig()
|
|
|
|
// Unless command line overrides, we use localhost for the server
|
|
if BaseUrl == "" {
|
|
Config.BaseUrl = "http://localhost:" + strconv.Itoa(serverPort)
|
|
}
|
|
|
|
build(serverWatch)
|
|
|
|
// Watch runs its own server as part of the routine
|
|
if serverWatch {
|
|
fmt.Println("Watching for changes in", Config.GetAbsPath(Config.ContentDir))
|
|
err := NewWatcher(serverPort)
|
|
if err != nil {
|
|
fmt.Println(err)
|
|
}
|
|
}
|
|
|
|
serve(serverPort)
|
|
}
|
|
|
|
func serve(port int) {
|
|
if Verbose {
|
|
fmt.Println("Serving pages from " + Config.GetAbsPath(Config.PublishDir))
|
|
}
|
|
|
|
fmt.Printf("Web Server is available at http://localhost:%v\n", port)
|
|
fmt.Println("Press ctrl+c to stop")
|
|
panic(http.ListenAndServe(":"+strconv.Itoa(port), http.FileServer(http.Dir(Config.GetAbsPath(Config.PublishDir)))))
|
|
}
|