mirror of
https://github.com/gohugoio/hugo.git
synced 2024-07-02 15:20:28 +00:00
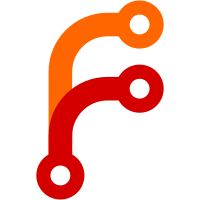
Add a new rssLimit site configuration option with default of 15. Prior to this fix, you could create your own RSS feed to override the default limit of 15, but we still had a hardcoded limit of 50 items set in `hugolib.renderRSS()`. With this option in place, the `range first 15 .Data.Pages` logic is no longer hardcoded into the embedded RSS template. Because the size of the slice passed to the template is now limited to rssLimit instead of 50, this commit is a breaking change for sites with a custom RSS template that expects more than 15 items. Fixes #3035
117 lines
3.9 KiB
Go
117 lines
3.9 KiB
Go
// Copyright 2016-present The Hugo Authors. All rights reserved.
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
package hugolib
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/spf13/afero"
|
|
"github.com/spf13/hugo/helpers"
|
|
"github.com/spf13/viper"
|
|
)
|
|
|
|
// LoadConfig loads Hugo configuration into a new Viper and then adds
|
|
// a set of defaults.
|
|
func LoadConfig(fs afero.Fs, relativeSourcePath, configFilename string) (*viper.Viper, error) {
|
|
v := viper.New()
|
|
v.SetFs(fs)
|
|
if relativeSourcePath == "" {
|
|
relativeSourcePath = "."
|
|
}
|
|
|
|
v.AutomaticEnv()
|
|
v.SetEnvPrefix("hugo")
|
|
v.SetConfigFile(configFilename)
|
|
// See https://github.com/spf13/viper/issues/73#issuecomment-126970794
|
|
if relativeSourcePath == "" {
|
|
v.AddConfigPath(".")
|
|
} else {
|
|
v.AddConfigPath(relativeSourcePath)
|
|
}
|
|
err := v.ReadInConfig()
|
|
if err != nil {
|
|
if _, ok := err.(viper.ConfigParseError); ok {
|
|
return nil, err
|
|
}
|
|
return nil, fmt.Errorf("Unable to locate Config file. Perhaps you need to create a new site.\n Run `hugo help new` for details. (%s)\n", err)
|
|
}
|
|
|
|
v.RegisterAlias("indexes", "taxonomies")
|
|
|
|
loadDefaultSettingsFor(v)
|
|
|
|
return v, nil
|
|
}
|
|
|
|
func loadDefaultSettingsFor(v *viper.Viper) {
|
|
|
|
c := helpers.NewContentSpec(v)
|
|
|
|
v.SetDefault("cleanDestinationDir", false)
|
|
v.SetDefault("watch", false)
|
|
v.SetDefault("metaDataFormat", "toml")
|
|
v.SetDefault("disable404", false)
|
|
v.SetDefault("disableRSS", false)
|
|
v.SetDefault("disableSitemap", false)
|
|
v.SetDefault("disableRobotsTXT", false)
|
|
v.SetDefault("contentDir", "content")
|
|
v.SetDefault("layoutDir", "layouts")
|
|
v.SetDefault("staticDir", "static")
|
|
v.SetDefault("archetypeDir", "archetypes")
|
|
v.SetDefault("publishDir", "public")
|
|
v.SetDefault("dataDir", "data")
|
|
v.SetDefault("i18nDir", "i18n")
|
|
v.SetDefault("themesDir", "themes")
|
|
v.SetDefault("defaultLayout", "post")
|
|
v.SetDefault("buildDrafts", false)
|
|
v.SetDefault("buildFuture", false)
|
|
v.SetDefault("buildExpired", false)
|
|
v.SetDefault("uglyURLs", false)
|
|
v.SetDefault("verbose", false)
|
|
v.SetDefault("ignoreCache", false)
|
|
v.SetDefault("canonifyURLs", false)
|
|
v.SetDefault("relativeURLs", false)
|
|
v.SetDefault("removePathAccents", false)
|
|
v.SetDefault("taxonomies", map[string]string{"tag": "tags", "category": "categories"})
|
|
v.SetDefault("permalinks", make(PermalinkOverrides, 0))
|
|
v.SetDefault("sitemap", Sitemap{Priority: -1, Filename: "sitemap.xml"})
|
|
v.SetDefault("defaultExtension", "html")
|
|
v.SetDefault("pygmentsStyle", "monokai")
|
|
v.SetDefault("pygmentsUseClasses", false)
|
|
v.SetDefault("pygmentsCodeFences", false)
|
|
v.SetDefault("pygmentsOptions", "")
|
|
v.SetDefault("disableLiveReload", false)
|
|
v.SetDefault("pluralizeListTitles", true)
|
|
v.SetDefault("preserveTaxonomyNames", false)
|
|
v.SetDefault("forceSyncStatic", false)
|
|
v.SetDefault("footnoteAnchorPrefix", "")
|
|
v.SetDefault("footnoteReturnLinkContents", "")
|
|
v.SetDefault("newContentEditor", "")
|
|
v.SetDefault("paginate", 10)
|
|
v.SetDefault("paginatePath", "page")
|
|
v.SetDefault("blackfriday", c.NewBlackfriday())
|
|
v.SetDefault("rSSUri", "index.xml")
|
|
v.SetDefault("rssLimit", 15)
|
|
v.SetDefault("sectionPagesMenu", "")
|
|
v.SetDefault("disablePathToLower", false)
|
|
v.SetDefault("hasCJKLanguage", false)
|
|
v.SetDefault("enableEmoji", false)
|
|
v.SetDefault("pygmentsCodeFencesGuessSyntax", false)
|
|
v.SetDefault("useModTimeAsFallback", false)
|
|
v.SetDefault("defaultContentLanguage", "en")
|
|
v.SetDefault("defaultContentLanguageInSubdir", false)
|
|
v.SetDefault("enableMissingTranslationPlaceholders", false)
|
|
v.SetDefault("enableGitInfo", false)
|
|
}
|