mirror of
https://github.com/gohugoio/hugo.git
synced 2024-06-27 21:25:07 +00:00
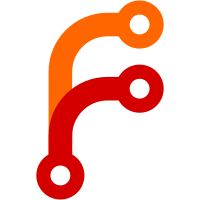
canonifyUrls=true, RelPermalink and baseUrl with sub-path did not work. This fixes that by adding a check for canonifyUrl=trues=true in RelPermalink(). So given - baseUrl "http://somehost.com/sub/" - the path "some-path/file.html" For canonifyUrls=false RelPermalink() returns "/sub/some-path/file.html" For canonifyUrls=true RelPermalink() returns "/some-path/file.html" In the last case, the Url will be made absolute and clickable in a later step. This commit also makes the menu urls defined in site config releative. To make them work with canonifying of urls, the context root is prepended if canonifying is turned off. Fixes #519 Fixes #711
87 lines
3 KiB
Go
87 lines
3 KiB
Go
package hugolib
|
|
|
|
import (
|
|
"html/template"
|
|
"path/filepath"
|
|
"testing"
|
|
|
|
"github.com/spf13/hugo/source"
|
|
"github.com/spf13/viper"
|
|
)
|
|
|
|
func TestPermalink(t *testing.T) {
|
|
tests := []struct {
|
|
file string
|
|
dir string
|
|
base template.URL
|
|
slug string
|
|
url string
|
|
uglyUrls bool
|
|
canonifyUrls bool
|
|
expectedAbs string
|
|
expectedRel string
|
|
}{
|
|
{"x/y/z/boofar.md", "x/y/z", "", "", "", false, false, "/x/y/z/boofar/", "/x/y/z/boofar/"},
|
|
{"x/y/z/boofar.md", "x/y/z/", "", "", "", false, false, "/x/y/z/boofar/", "/x/y/z/boofar/"},
|
|
{"x/y/z/boofar.md", "x/y/z/", "", "boofar", "", false, false, "/x/y/z/boofar/", "/x/y/z/boofar/"},
|
|
{"x/y/z/boofar.md", "x/y/z", "http://barnew/", "", "", false, false, "http://barnew/x/y/z/boofar/", "/x/y/z/boofar/"},
|
|
{"x/y/z/boofar.md", "x/y/z/", "http://barnew/", "boofar", "", false, false, "http://barnew/x/y/z/boofar/", "/x/y/z/boofar/"},
|
|
{"x/y/z/boofar.md", "x/y/z", "", "", "", true, false, "/x/y/z/boofar.html", "/x/y/z/boofar.html"},
|
|
{"x/y/z/boofar.md", "x/y/z/", "", "", "", true, false, "/x/y/z/boofar.html", "/x/y/z/boofar.html"},
|
|
{"x/y/z/boofar.md", "x/y/z/", "", "boofar", "", true, false, "/x/y/z/boofar.html", "/x/y/z/boofar.html"},
|
|
{"x/y/z/boofar.md", "x/y/z", "http://barnew/", "", "", true, false, "http://barnew/x/y/z/boofar.html", "/x/y/z/boofar.html"},
|
|
{"x/y/z/boofar.md", "x/y/z/", "http://barnew/", "boofar", "", true, false, "http://barnew/x/y/z/boofar.html", "/x/y/z/boofar.html"},
|
|
{"x/y/z/boofar.md", "x/y/z/", "http://barnew/boo/", "boofar", "", true, false, "http://barnew/boo/x/y/z/boofar.html", "/boo/x/y/z/boofar.html"},
|
|
{"x/y/z/boofar.md", "x/y/z/", "http://barnew/boo/", "boofar", "", true, true, "http://barnew/boo/x/y/z/boofar.html", "/x/y/z/boofar.html"},
|
|
{"x/y/z/boofar.md", "x/y/z/", "http://barnew/boo", "boofar", "", true, true, "http://barnew/boo/x/y/z/boofar.html", "/x/y/z/boofar.html"},
|
|
|
|
// test url overrides
|
|
{"x/y/z/boofar.md", "x/y/z", "", "", "/z/y/q/", false, false, "/z/y/q/", "/z/y/q/"},
|
|
}
|
|
|
|
viper.Set("DefaultExtension", "html")
|
|
|
|
for i, test := range tests {
|
|
viper.Set("uglyurls", test.uglyUrls)
|
|
viper.Set("canonifyurls", test.canonifyUrls)
|
|
p := &Page{
|
|
Node: Node{
|
|
UrlPath: UrlPath{
|
|
Section: "z",
|
|
Url: test.url,
|
|
},
|
|
Site: &SiteInfo{
|
|
BaseUrl: test.base,
|
|
},
|
|
},
|
|
Source: Source{File: *source.NewFile(filepath.FromSlash(test.file))},
|
|
}
|
|
|
|
if test.slug != "" {
|
|
p.update(map[string]interface{}{
|
|
"slug": test.slug,
|
|
})
|
|
}
|
|
|
|
u, err := p.Permalink()
|
|
if err != nil {
|
|
t.Errorf("Test %d: Unable to process permalink: %s", i, err)
|
|
}
|
|
|
|
expected := test.expectedAbs
|
|
if u != expected {
|
|
t.Errorf("Test %d: Expected abs url: %s, got: %s", i, expected, u)
|
|
}
|
|
|
|
u, err = p.RelPermalink()
|
|
if err != nil {
|
|
t.Errorf("Test %d: Unable to process permalink: %s", i, err)
|
|
}
|
|
|
|
expected = test.expectedRel
|
|
if u != expected {
|
|
t.Errorf("Test %d: Expected rel url: %s, got: %s", i, expected, u)
|
|
}
|
|
}
|
|
}
|